Set an Element’s Height Equal to the Window’s Height
A simple, responsive way to set the height of an element to the height of the browser window.
Update: This can now be done with the CSS vh unit, which is relative to the viewport.
.sidebar { height: 100vh; }
Typically CSS handles the layout of a page, but it’s hard to make the height of a floated element fill the viewport without extra markup.
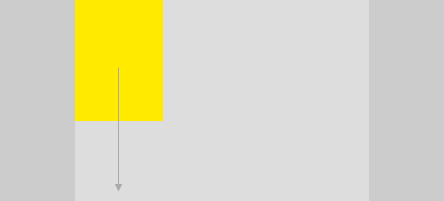
Instead of adding extra markup or CSS hacks, JavaScript can find the window height and set an element height so it fills the window vertically and changes height when the browser window is resized.
I create a function to save and set the height. The function runs when the page loads, and it runs again when the viewport is resized.
Within the function JavaScript queries the DOM for .sidebar
and sets the CSS min-height
property to the innerHeight()
of the browser window. In most cases it’s probably best to specify min-height
, so the elements height can increase when needed, that way nothing gets cut-off if the content extends past the viewport.
$(document).ready(function() {
function setHeight() {
windowHeight = $(window).innerHeight();
$('.sidebar').css('min-height', windowHeight);
};
setHeight();
$(window).resize(function() {
setHeight();
});
});
Just replace .sidebar
with the class or id of the element you wish to target.
This example relies on jQuery methods, so the library needs to be included if it isn’t already. For a vanilla JavaScript solution, checkout You Might Not Need jQuery.